Overview
UART (Universal Asynchronous Receiver Transmitter) is a serial communication protocol commonly used in microcontrollers for transmitting and receiving data. The STM32 microcontroller series from STMicroelectronics provides UART hardware modules that can be easily configured and used for serial communication.
Configuration
Baud Rate
The baud rate determines the speed at which data is transmitted over the UART communication channel. It specifies the number of data bits transmitted per second. The STM32 microcontrollers support a wide range of baud rates, typically ranging from a few hundred to several megabits per second.
Data Bits
The number of data bits in each UART frame can be configured to be 7, 8, or 9 bits. Most commonly, 8 data bits are used, providing a range of 256 unique characters to be transmitted.
Parity Bits
Parity bits are optional error-checking bits that can be included in each UART frame. They can be used to detect and correct transmission errors. The parity bit can be configured as even, odd, or disabled.
Stop Bits
Stop bits indicate the end of a UART frame. The most commonly used stop bit configuration is one stop bit. However, two stop bits can also be used for more reliable transmission in noisy environments.
Initialization
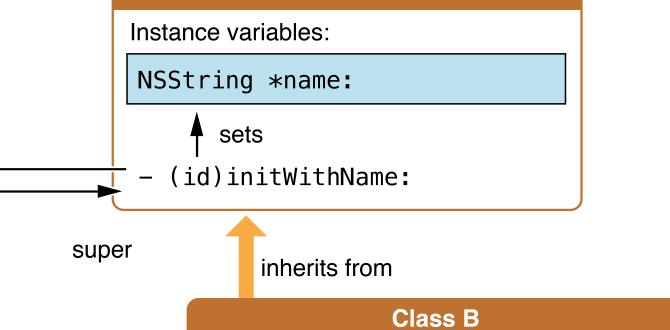
Enable UART Peripheral
Firstly, the UART peripheral on the STM32 microcontroller needs to be enabled. This can be done by setting the corresponding enable bit in the UART control register.
Set UART Parameters
The desired UART parameters such as baud rate, data bits, parity bits, and stop bits need to be configured. This can be done by writing the appropriate values to the corresponding registers.
Initialize GPIO Pins
The GPIO (General Purpose Input Output) pins corresponding to the UART peripheral need to be initialized. This involves configuring the pins as alternate function output to enable UART communication.
Configure Interrupts
If interrupt-driven communication is desired, interrupts can be enabled for UART receive and transmit events. Interrupt handlers can be defined to handle these events asynchronously.
Transmitting Data
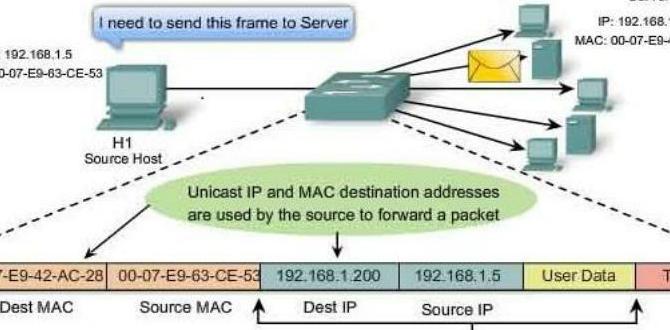
Write to UART Data Register
To transmit data over UART, the data needs to be written to the UART data register. The data will be automatically transmitted when the UART is ready for transmission.
Interrupt-Driven Transmission
In interrupt-driven transmission, the UART interrupt handler can be used to transmit data in the background while the microcontroller performs other tasks. The transmit interrupt flag needs to be monitored to ensure the UART is ready for the next transmission.
DMA-Driven Transmission
DMA (Direct Memory Access) can be used to offload data transmission from the CPU. DMA allows data to be transferred directly from memory to the UART data register without CPU intervention. This can be useful for high-speed data transfer.
Receiving Data
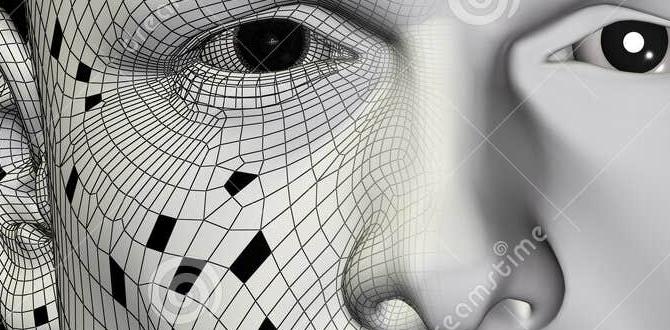
Read from UART Data Register
To receive data over UART, the data needs to be read from the UART data register. The received data can be stored in a buffer for further processing.
Interrupt-Driven Reception
In interrupt-driven reception, the UART interrupt handler can be used to receive data in the background while the microcontroller performs other tasks. The receive interrupt flag needs to be monitored to handle incoming data.
DMA-Driven Reception
DMA can also be used for receiving data. DMA can automatically transfer received data from the UART data register to a memory buffer without CPU intervention. This can be useful for handling large amounts of incoming data.
Error Handling
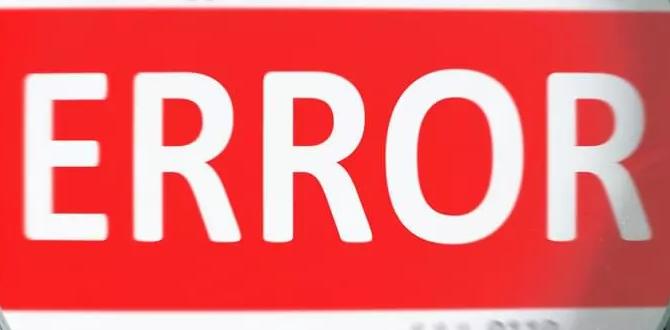
Overflow Error
If the incoming data rate is higher than the microcontroller can handle, an overflow error may occur. This can be detected by monitoring the overflow flag and appropriate error handling mechanisms can be implemented.
Frame Error
A frame error occurs when the received data frame is not valid. This can be caused by noise on the communication line or incorrect UART settings. Frame errors can be detected by monitoring the frame error flag.
Parity Error
A parity error occurs when the received data frame parity does not match the expected parity. This can be used to detect and correct transmission errors. Parity errors can be detected by monitoring the parity error flag.
Sample Code
Here is a sample code snippet to demonstrate UART initialization and transmission using the STM32 microcontroller:“`c#include “stm32f4xx.h”void UART_Init(){ // Enable UART2 clock RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE); USART_InitTypeDef USART_InitStruct; // Configure UART parameters USART_InitStruct.USART_BaudRate = 9600; USART_InitStruct.USART_WordLength = USART_WordLength_8b; USART_InitStruct.USART_StopBits = USART_StopBits_1; USART_InitStruct.USART_Parity = USART_Parity_No; USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None; USART_InitStruct.USART_Mode = USART_Mode_Tx; // Initialize UART2 USART_Init(USART2, &USART_InitStruct); // Enable UART2 USART_Cmd(USART2, ENABLE);}void UART_SendChar(char c){ // Wait until UART2 is ready for transmission while (!(USART2->SR & USART_SR_TXE)); // Send character USART_SendData(USART2, c);}int main(){ // Initialize UART2 UART_Init(); // Send “Hello, World!” over UART char message[] = “Hello, World!”; for (int i = 0; i < sizeof(message)-1; i++) { UART_SendChar(message[i]); } while (1) { // Main loop }}```Conclusion
UART communication is a crucial aspect of microcontroller applications. The STM32 microcontrollers provide flexible and easy-to-use UART modules that can be configured for various communication requirements. By understanding the configuration, initialization, and transmission/reception methods, developers can utilize UART effectively in their STM32 projects.
Frequently Asked Questions
Q1: Is UART the same as USART?A1: UART (Universal Asynchronous Receiver Transmitter) and USART (Universal Synchronous Asynchronous Receiver Transmitter) are similar in functionality but differ in synchronization. UART is asynchronous, while USART can operate in both synchronous and asynchronous modes. Q2: How do I choose the appropriate baud rate for my UART communication?
A2: The baud rate should be chosen based on the required data transfer rate and the capabilities of the receiving device. It is important to ensure that both the transmitting and receiving devices are set to the same baud rate for successful communication.Q3: What is the purpose of parity bits in UART communication?
A3: Parity bits provide a simple error checking mechanism in UART communication. They can detect transmission errors caused by noise and ensure the integrity of the transmitted data.Q4: Can I use UART for long-distance communication?
A4: UART is suitable for short-distance communication due to its limited noise immunity. For long-distance communication, additional measures like voltage level translation and noise suppression techniques may be required.Q5: Can I use multiple UART modules in an STM32 microcontroller?
A5: Yes, STM32 microcontrollers often include multiple UART modules that can be used simultaneously. Each UART module can be configured independently for different communication requirements.